Quick and easy metrics notifier
With Interval you can:
- Send email or Slack notifications from actions
- Schedule actions to be run on a recurring basis
Since Interval already works alongside your database and backend, these features in combination make it trivial to quickly spin up reminders to keep your team aware of important metrics. You can even send notifications on a particular threshold or condition, which is useful to maintain data integrity and alert when expectations are not met.
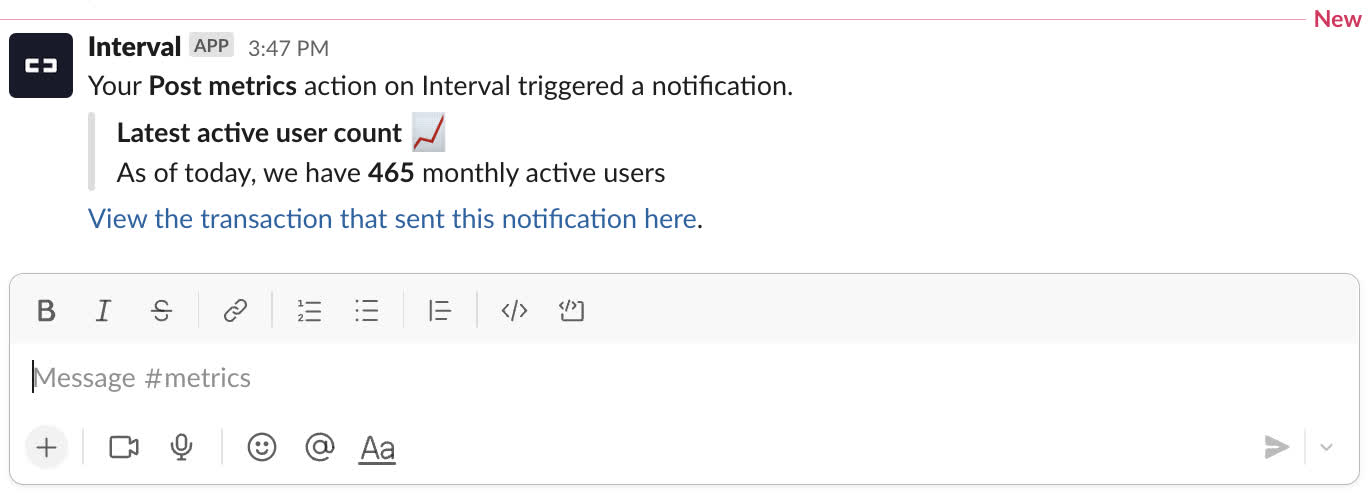
How it works
This example uses Prisma to query our PostgreSQL database, but you can easily swap these out for your own metrics with whatever you typically use to query your datastore.
First, we'll add our queries to a basic Interval action and simply log the output. When building tools with Interval, it's often useful to leverage ctx.log
for debugging and getting a better idea of the data available to you.
import { Action, io } from "@interval/sdk";
import { PrismaClient } from "@prisma/client";
const prisma = new PrismaClient();
export async function activeUsers() {
return await prisma.$queryRaw`SELECT count(*) FROM "Users" WHERE active = true`;
}
export default new Action(async () => {
const count = await activeUsers();
ctx.log("Active user count:", count);
});
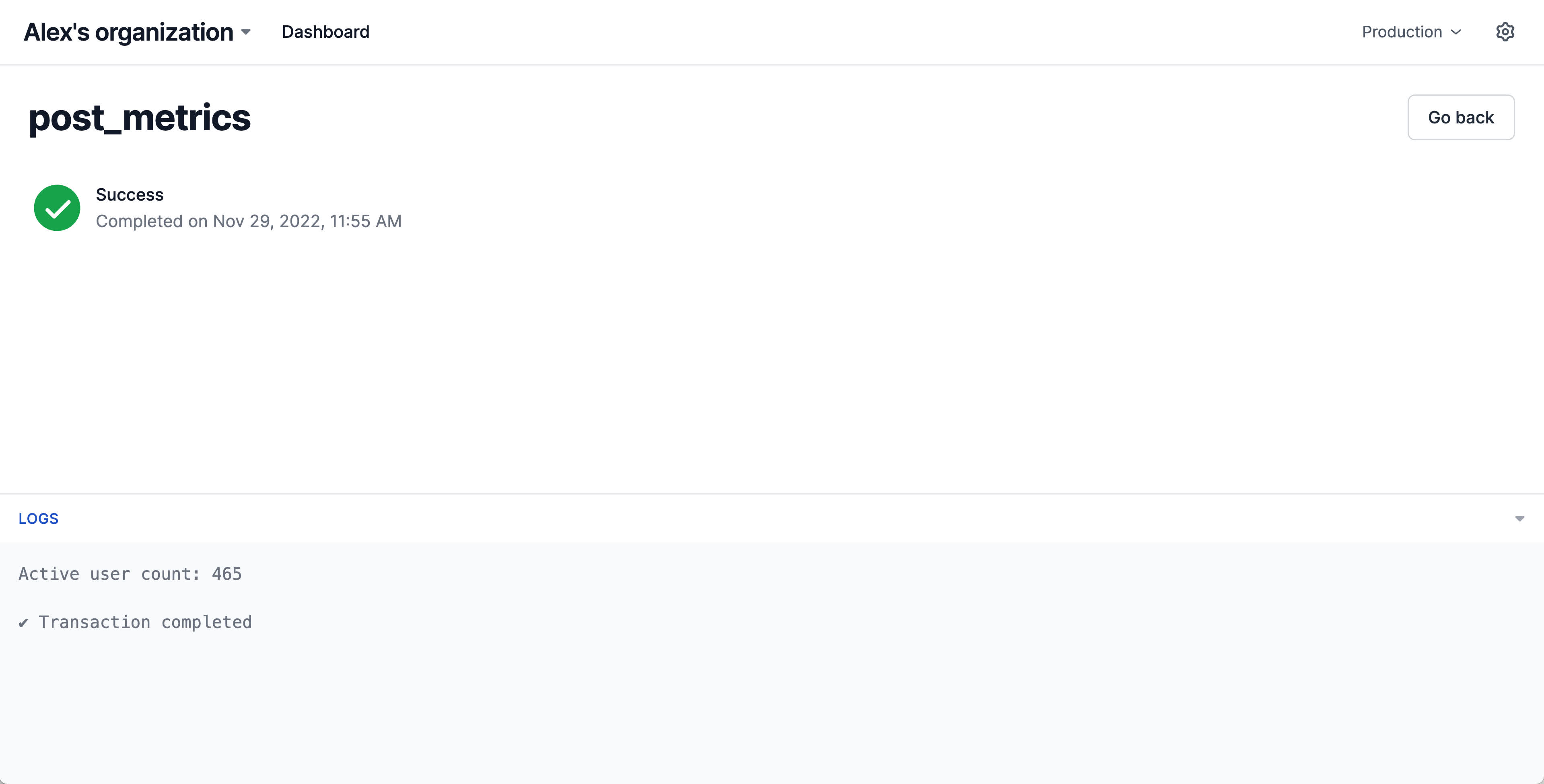
Next we'll make a call to ctx.notify
to post the query results to our team's slack channel.
info
To send Slack notifications, you'll need to:
- Connect your organization to your Slack workspace
- Add the Interval Slack app to any channels you'd like to post to :::
import { Action, ctx } from "@interval/sdk";
import { PrismaClient } from "@prisma/client";
const prisma = new PrismaClient();
export async function activeUsers() {
return await prisma.$queryRaw`SELECT count(*) FROM "Users" WHERE active = true`;
}
export default new Action(async () => {
const count = await activeUsers();
ctx.log("Active user count:", count);
await ctx.notify({
message: `As of today, we have *${count}* monthly active users`,
title: "Latest active user count 📈",
delivery: [
{
to: "#metrics",
method: "SLACK",
},
],
});
});
Now, each time the action runs, Interval will send off the results of your query to post to the specified Slack channel.
Notifications are not delivered for actions running in the Development environment with your personal development key. To test notifications for real, you'll have to deploy your action to production. :::
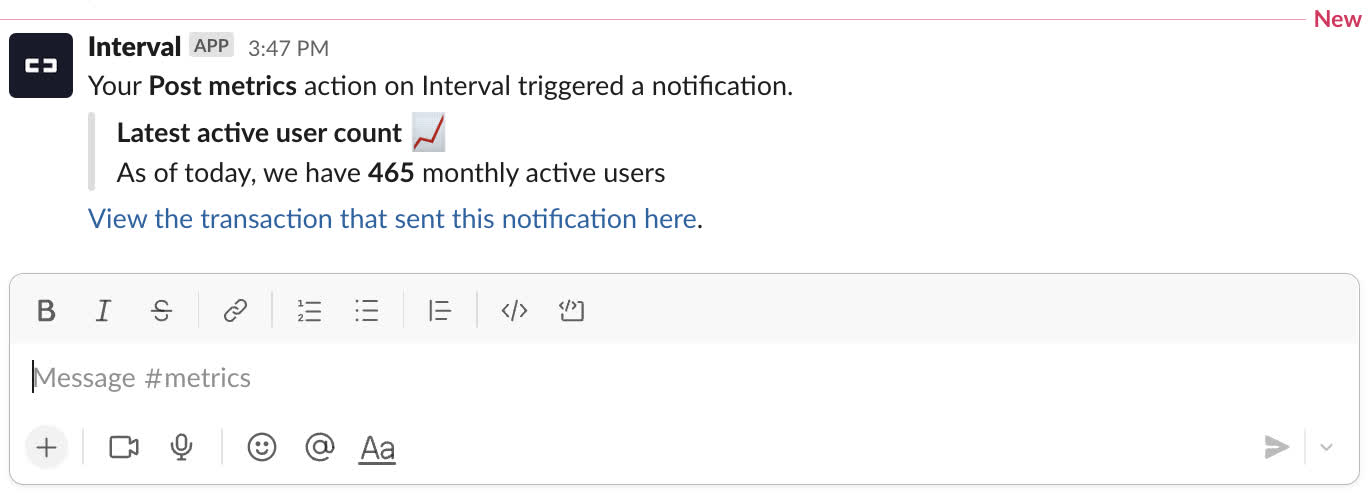
Next, we'll schedule the action to run automatically at a set time. To add a schedule, we first need to configure our action to run in the background. You can update this setting in the dashboard or you can configure the backgroundable
property in your action definition code.
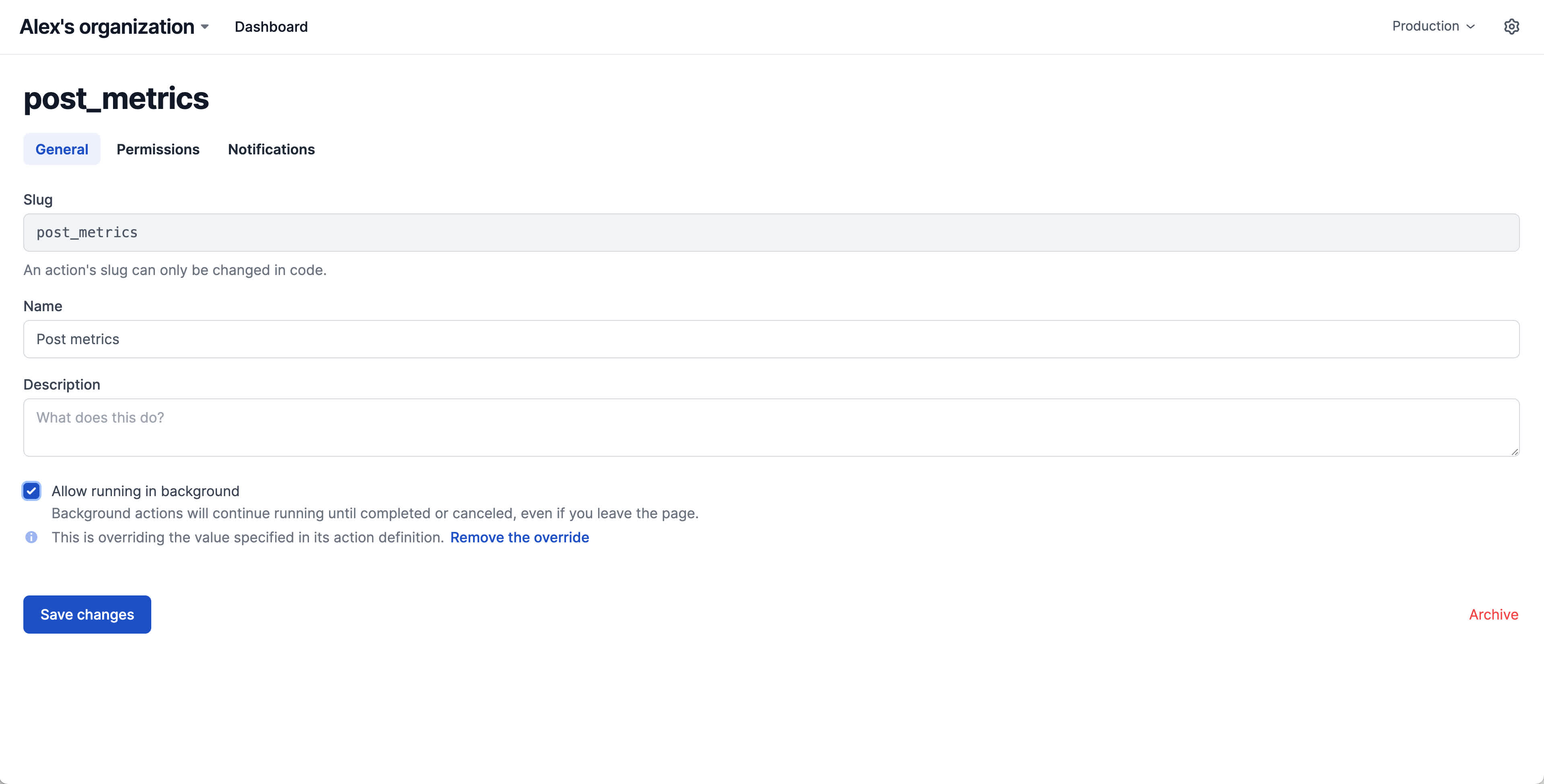
import { Action, ctx } from "@interval/sdk";
import { PrismaClient } from "@prisma/client";
const prisma = new PrismaClient();
export async function activeUsers() {
return await prisma.$queryRaw`SELECT count(*) FROM "Users" WHERE active = true`;
}
export default new Action({
backgroundable: true,
handler: async () => {
const count = await activeUsers();
ctx.log("Active user count:", count);
await ctx.notify({
message: `As of today, we have *${count}* monthly active users`,
title: "Latest active user count 📈",
delivery: [
{
to: "#metrics",
method: "SLACK",
},
],
});
},
});
Once the action is backgroundable, a recurring schedule can be set on the action's settings page in the Interval dashboard.
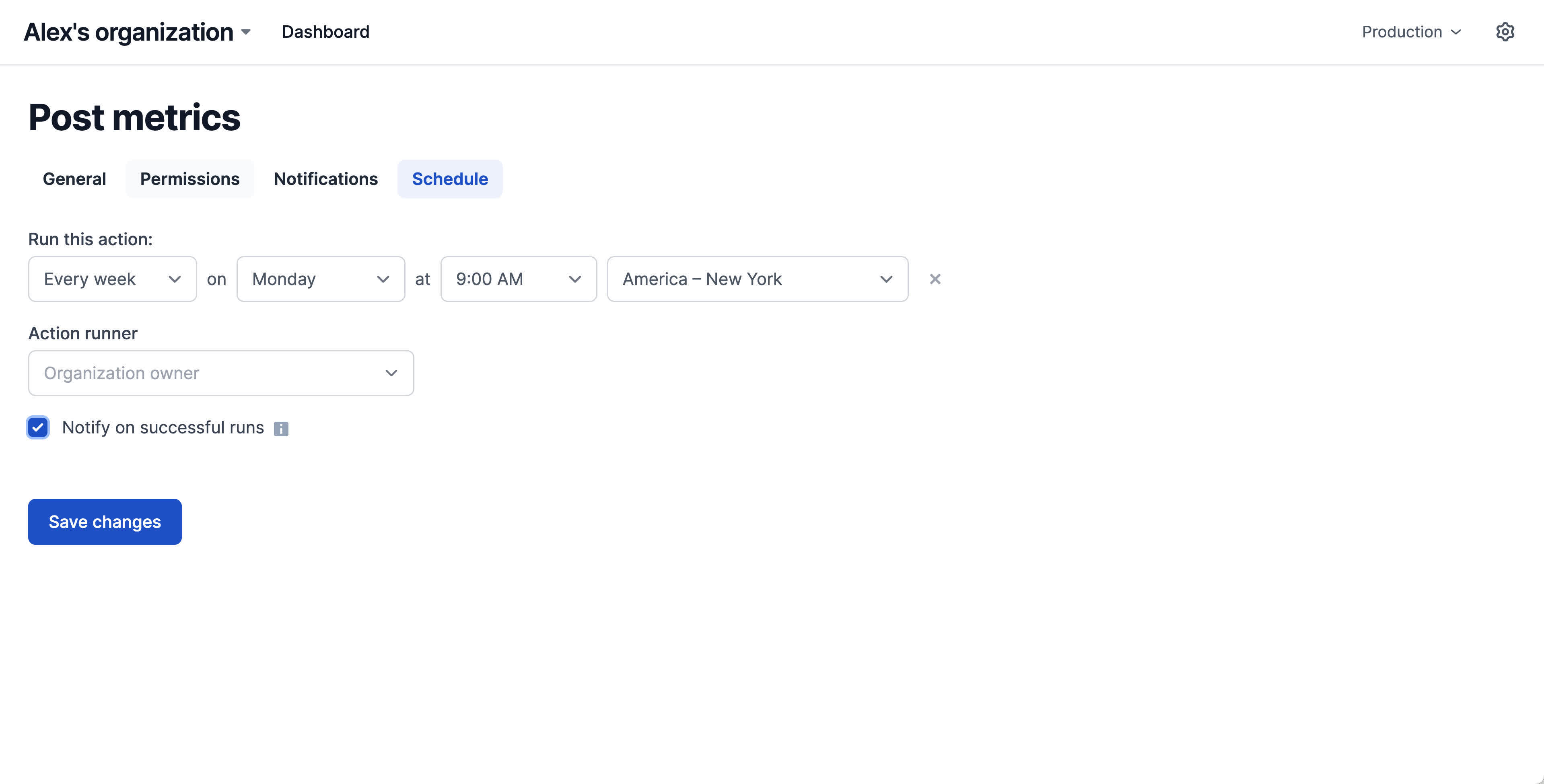
The full source code is available for this example.